To allow your xApp to call the XUMM API's from your xApp frontend without the need for your own backend or proxy, use the xApp JWT endpoints.
If you are building an xApp and the xApp already requires your own backend (e.g. to persist data, fetch user info, etc.) you can use the regular XUMM Backend API.
However, if you are building an xApp and want to communicate straight from your xApp frontend with the XUMM platform, you can use the xApp JWT endpoints (documented here).
The xApp JWT endpoints allow you to turn an xApp 'OTT' (One Time Token) into a JWT (Json Web Token, see https://jwt.io). The obtained JWT can then be used for 24 hours to communicate directly from your xApp frontend using client side Javascript to the XUMM platform backend.
Option #1: Use the XUMM SDK in your xApp frontend
The XUMM SDK offers browserified frontend JWT flow support out of the box since version 1.0. The browserified XUMM SDK is available on the jsDeliver CDN:
<script src="https://cdn.jsdelivr.net/npm/xumm-sdk/dist/browser.min.js"></script>
XUMM will call your xApp with the URL Query Param. xAppToken
appended. The XUMM SDK will pick up on this param. automatically, so all you have to provide is your (public) API Key (get it at the XUMM Developer Console).
After loading the XUMM SDK using the HTML above, all you need to do to fetch your OTT data & make calls to the XUMM platform is:
const {XummSdkJwt} = require('xumm-sdk')
const Sdk = new XummSdkJwt('8525e32b-1bd0-4839-af2f-f794874a80b0')
Sdk.getOttData().then(c => {
console.log('OTT Data', c)
Sdk.ping().then(c => {
console.log('Pong', c)
})
})
Here's a sample JSFiddle.
Option #2: Build your own JWT flow
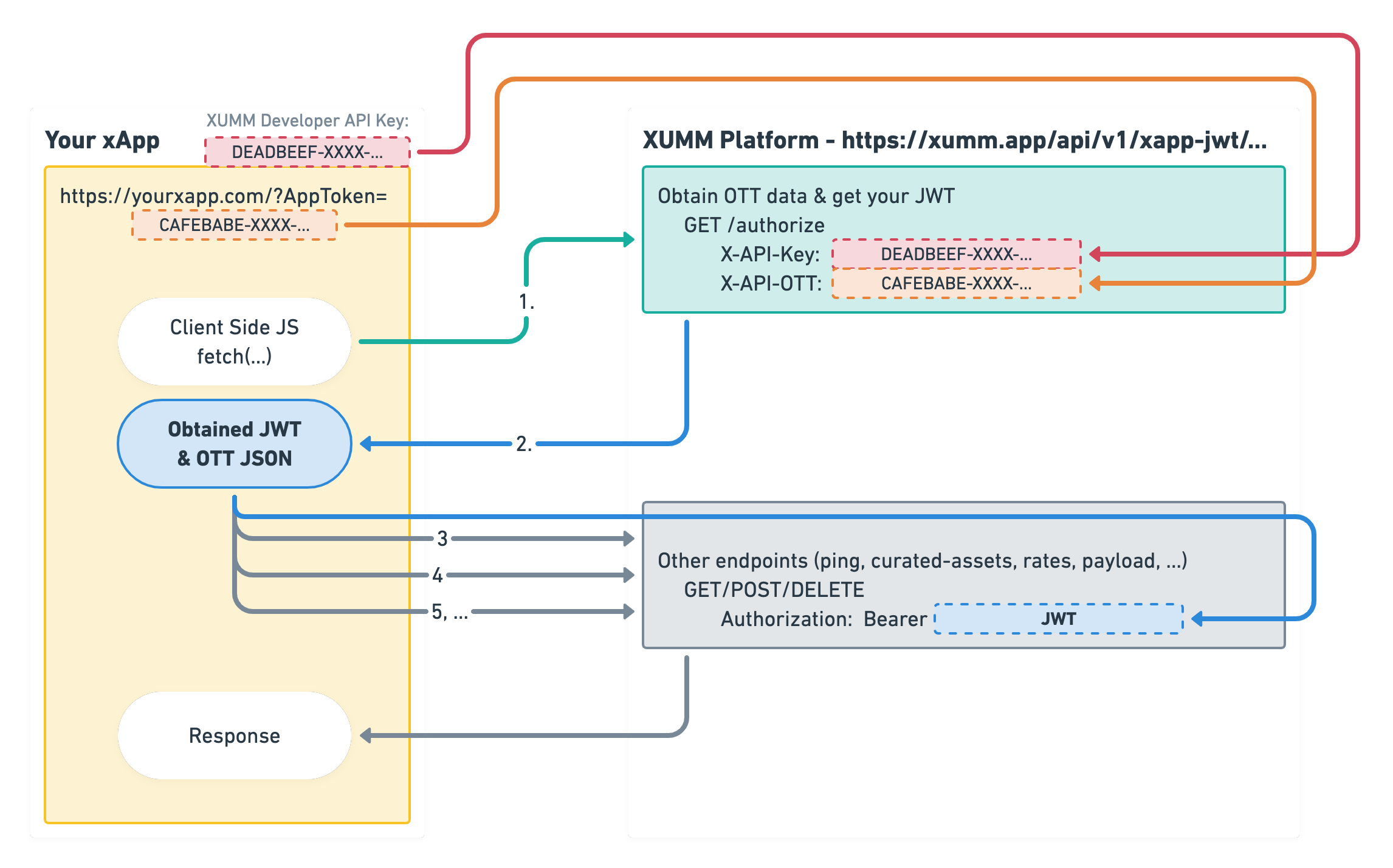
OTT: One Time Token
Once an OTT has been used to call the authorize endpoint and a JWT has been provided, the OTT expired and can't be used to call the authorize endpoint unless if you are the developer, see: Development: OTT re-fetching